队列:只允许在一端进行插入,在另一端进行删除的线性表
链队列:使用链表实现的队列,具有队头指针和队尾指针,指示队列元素所在的位置
链队列特性:
●只能队尾插入元素,在队头删除元素
●先进先出(First In First Out)的线性表,先进入的元素出队,后进入的元素才能出队
队列示意图:
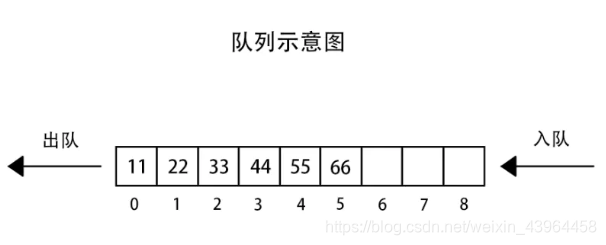
空链队列:
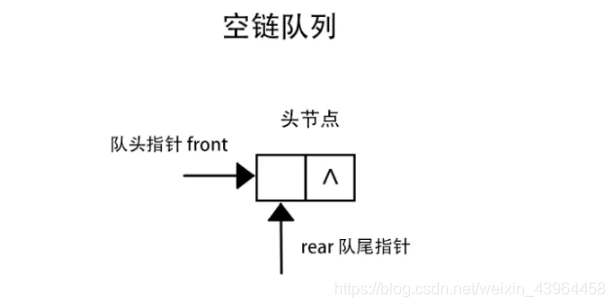
链队的入栈操作:
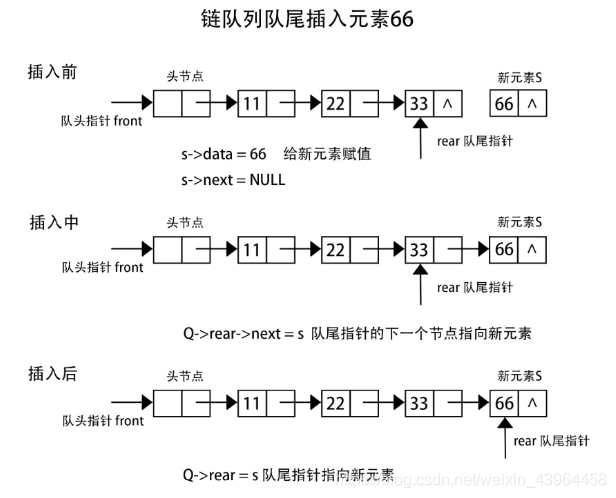
链队的出栈操作:
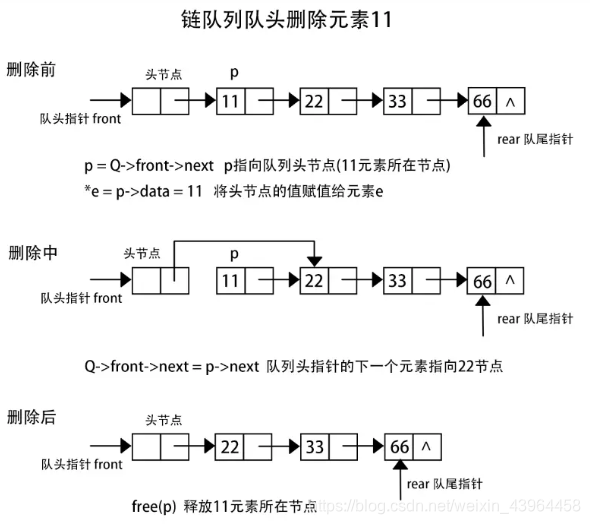
————————纸上得来终觉浅,绝知此事要躬行————————
话不多说,把代码开起来,这样晦涩的概念才不会显得苍白无力!!!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157
| #include<stdio.h> #include<malloc.h> #include<assert.h>
typedef int ElemType;
typedef struct QueueNode { ElemType data; struct QueueNode *next; }QueueNode,*PNode;
typedef struct LinkQueue { PNode front; PNode tail; }LinkQueue;
void InitQueue(LinkQueue *Q) {
QueueNode *s=(QueueNode *)malloc(sizeof(QueueNode)); assert(s!=NULL); Q->front=Q->tail=s; Q->tail->next=NULL; }
void EnQueue(LinkQueue *Q,ElemType x) { QueueNode *s=(QueueNode *)malloc(sizeof(QueueNode)); assert(s!=NULL); s->data=x; s->next=NULL; Q->tail->next=s; Q->tail=s; }
void ShowQueue(LinkQueue *Q) { QueueNode *p=Q->front->next; printf("Front:>"); while(p!=NULL) { printf("%d ",p->data); p=p->next; } printf("<:Tail\n"); }
void DenQueue(LinkQueue *Q) {
if(Q->front==Q->tail) return; QueueNode *p=Q->front->next; Q->front->next=p->next; free(p); if(p==Q->tail) Q->tail=Q->front; }
void GetHead(LinkQueue *Q,ElemType *v) { if(Q->front==Q->tail) return; QueueNode *p=Q->front->next; *v=p->data; }
int Length(LinkQueue *Q) { int len=0; QueueNode *p=Q->front->next; while(p!=NULL) { len++; p=p->next; } return len; }
void ClearQueue(LinkQueue *Q) { if(Q->front==Q->tail) return; QueueNode *p=Q->front->next; while(p!=NULL) { Q->front->next=p->next; free(p); p=Q->front->next; }
Q->tail=Q->front; }
void DestroyQueue(LinkQueue *Q) { ClearQueue(Q); free(Q->front); Q->front=Q->tail=NULL; }
void main() { LinkQueue Q; ElemType e; InitQueue(&Q); for(int i=1;i<=10;++i) { EnQueue(&Q,i); } ShowQueue(&Q); printf("出队\n"); DenQueue(&Q); GetHead(&Q,&e); printf("链队头元素为:%d\n",e); ShowQueue(&Q); printf("链队的长度为:%d\n",Length(&Q)); printf("\n"); printf("出队\n"); DenQueue(&Q); GetHead(&Q,&e); printf("链队头元素为:%d\n",e); ShowQueue(&Q); printf("链队的长度为:%d\n",Length(&Q)); printf("\n"); printf("出队\n"); DenQueue(&Q); GetHead(&Q,&e); printf("链队头元素为:%d\n",e); ShowQueue(&Q); printf("链队的长度为:%d\n",Length(&Q)); printf("\n"); printf("出队\n"); DenQueue(&Q); GetHead(&Q,&e); printf("链队头元素为:%d\n",e); ShowQueue(&Q); printf("链队的长度为:%d\n",Length(&Q)); printf("\n"); ClearQueue(&Q); DestroyQueue(&Q); }
|
运行结果:
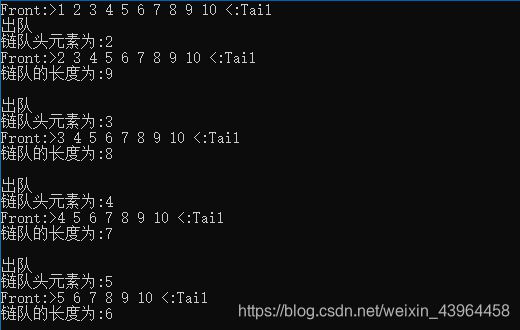