栈是限定仅在表面进行插入或删除操作的线性表。因此,对栈来说,表尾端有其特殊含义,称为栈顶,相应地,表头端称为栈底,不含元素的空表称为空栈。
顺序栈,即栈的顺序存储结构是利用一组地址连续的存储单元依次存放自栈底到栈顶的数据元素,同时附设指针top指示栈顶元素在顺序栈中的位置,通常的习惯做法是以top=0表示空栈。
话不多说,把代码跑起来!!!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129
| #include <stdio.h> #include <malloc.h> #include <assert.h>
typedef int ElemType; #define STACK_INIT_SIZE 8
typedef struct SeqStack { ElemType *base; int capacity; int top; }SeqStack;
void InitStack(SeqStack *s) {
s->base=(ElemType *)malloc(sizeof(ElemType)*STACK_INIT_SIZE); assert(s->base!=NULL); s->capacity=STACK_INIT_SIZE; s->top=0; }
bool IsFull(SeqStack *s) { return s->top >= s->capacity; }
bool IsEmpty(SeqStack *s) { return s->top==0; }
void Push(SeqStack *s,ElemType x) { if(IsFull(s)) { printf("栈空间已满,%d不能入栈\n",x); return; } s->base[s->top]=x; s->top++; }
void Pop(SeqStack *s) { if(IsEmpty(s)) { printf("栈空间已空,不能出栈\n"); return; } s->top--; }
bool GetTop(SeqStack *s,ElemType *v) { if(IsEmpty(s)) { printf("栈空间已空,不能取栈顶元素\n"); return false; } *v=s->base[s->top-1];
return true; }
void Show(SeqStack *s) { for(int i=s->top-1;i>=0;i--)
{ printf("%d\n",s->base[i]); } printf("\n"); }
int Length(SeqStack *s) { return s->top; }
void Clear(SeqStack *s) { s->top=0; }
void Destory(SeqStack *s) { free(s->base); s->base=NULL; s->capacity=s->top=0; }
void main() { SeqStack st; InitStack(&st); ElemType v; printf("入栈\n"); for(int i=1;i<=5;++i) { Push(&st,i); } Show(&st); printf("栈的长度为%d\n",Length(&st)); printf("\n"); GetTop(&st,&v); printf("%d 是栈顶元素\n",v); printf("\n"); printf("出栈\n"); Pop(&st); Show(&st); printf("栈的长度为%d\n",Length(&st)); printf("\n"); printf("清除栈\n"); Clear(&st); Show(&st); printf("栈的长度为%d\n",Length(&st)); Destory(&st); }
|
运行结果:
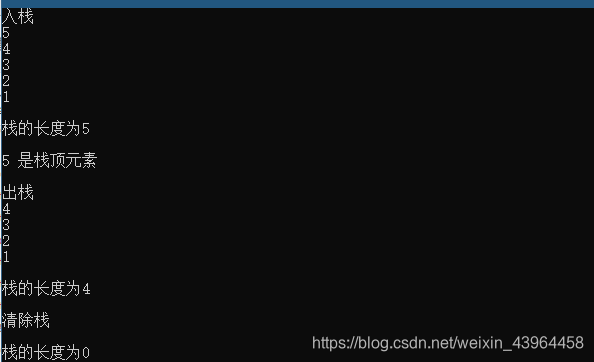